In previous post we already discuss that how to DevelopOfTheMethods in java. Now we are learning the syntax of a Method .
A syntax of the Java programming language is a set of rules defined and how a Java program is written and interpreted.
A syntax of methods mostly derived from C and C++. Unlike C++, in Java programming language there is no global functions or variables, but there are only data members which is also included as global variables.
In Java all code belongs to classes and all values are objects. Here only the exception is the primitive types, which is not represented by a instance class for performance reasons (though it can be automatically converted to objects and vice versa via auto-boxing). Some of the features like operator overloading or unsigned integer types are omitted to simplify the language and to avoid possible programming mistakes.
A syntax of the Java programming language is a set of rules defined and how a Java program is written and interpreted.
A syntax of methods mostly derived from C and C++. Unlike C++, in Java programming language there is no global functions or variables, but there are only data members which is also included as global variables.
In Java all code belongs to classes and all values are objects. Here only the exception is the primitive types, which is not represented by a instance class for performance reasons (though it can be automatically converted to objects and vice versa via auto-boxing). Some of the features like operator overloading or unsigned integer types are omitted to simplify the language and to avoid possible programming mistakes.
Syntax of a Method
1.Return Type 2. Method Name (3.list of formal parameters )
{
4.Block Of Statement's (or) Business Logic
}
Explanation
- Return Type represents in which type of value is return by the method. If any method does not returning any value then return type is "void". Void is one of the return type but not a data type.
- Method name represents the name of the method , In any Java programming method is containing either one or more words then we must have write "first word first letter must be small rest of the remaining words capital". This is also known "Hungarian rule" . Example:- getStudentDetails( );
- List Of Formal Parameters represents the variable names which is used for storing by the input , which is caring from the "Method call".
- Formal Parameter's is always used as the part of "Method Heading".
- Block of Statements represents the set of executable statements which is always providing solution for a client requirements and it is also known as "Business Logic".
- suppose if we use any variable inside the method body then we called as "Local Variable"
In java programming language, A Syntax's can be develop the methods by using 4 approaches . They are
1. A Method is taking input & returning values .
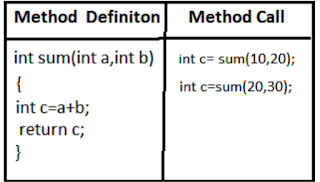
In the above example we have to take input on list of parameter as a method definition part and the values of a,b are given in the method call part.
2. A Method is non taking input & non returning values.

In the above example we do not take input on list of parameter's as a method definition part and the values of a,b are declared inside a method definition.
3. A Method is non taking input & returning values .

In the above example we do not take any input on the list of formal parameter's as a definition part and the values of a,b are declared inside in the method definition.
4. A Method is taking input & non returning values .

In the above example we have to take input on the list of formal parameter's as a definition part and the values of a,b are declared as a method call part.