A Sample java programs
Q. write a Java program which will display the sum of two number ?
program:
class Sum //Sub class
{
int a,b,c; // Data members
void set( ) // non - static methods
{
a=10;
b=20;
}
void sum( ) // Method Declaration
{
c=a+b;
}
void display( ) // Display the values
{
System.out.println("value of a="+a);
System.out.println("value of b="+b);
System.out.println("Sum="+c);
}
}//Business logic
class SumDemo // Mainclass
{
public static void main(String args[]) // Main Method
{
Sum s=new Sum( ); // Object declaration with sub class called Sum
s.set( );
s.sum( ); // methods callings
s.display( );
}
}// Execution logic
➩ Compile the above java program in command prompt.
cd> javac Sum.java ⤶
cd> java Sum ⤶
Output:- 30.
➩ Compile the above java program in command prompt.
cd> javac Sum.java ⤶
cd> java Sum ⤶
Output:- 30.
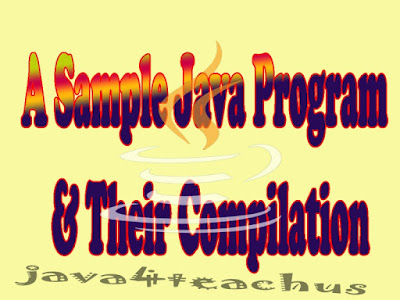
Q . write a Java program which will display the multiply of two number ?.
Program:
class Mul
{
int a,b,c;
void set()
{
a=10;
b=20;
}
void mul()
{
c=a*b;
}
void display()
{
System.out.println("value of a="+a);
System.out.println("value of b="+b);
System.out.println("multiplication="+c);
}}
class MulDemo
{
public static void main(String args[])
{
Mul m=new Mul();
m.set();
m.mul();
m.display();
}
}
Q . write a Java program which will display the "Division of two number" ?.
Program:-
class Division
{
int a,b,c;
void set()
{
a=4;
b=2;
}
void mul()
{
c=a/b;
}
void display()
{
System.out.println("value of a="+a);
System.out.println("value of b="+b);
System.out.println("multiplication="+c);
}}
class DivisionDemo
{
public static void main(String args[])
{
Division m=new Division();
m.set();
m.mul();
m.display();
}
}
Q. write a Java program which will display the "Area Of Triangle" ?.
Program :
class Triangle
{
int b,h,a;
void set();
{
b=1;
h=2;
}
void areaOfTriangle()
{
a = 0.5*b*h;
}
void display()
{
System.out.println("value of b="+b);
System.out.println("value of h="+h);
System.out.println("AreaOfTriangle="+a);
}
}
class TriangleDemo
{
public static void main(String args[])
{
Triangle t=new Triangle();
t.set();
t.areaOfTriangle();
t.display();
}
}
Q. write a Java program which will display the "PerimeterOfRectangle" ?.
program:
class PerimeterOfRectangle
{
int l,b,p;
void set()
{
l=10;
b=20;
}
void perimeterOfRectangle()
{
p=2*l*b;
}
void display()
{
System.out.println("value of l="+l);
System.out.println("value of b="+b);
System..out.println("perimeterofrectangle="+p);
}
}
class PerimeterDemo
{
public static void main(String args[])
{
PerimeterOfRectangle p= new PerimeterOfRectangle();
p.set();
p.perimeterOfRectangle();
p.display();
}
}
Q. write a Java program which will display the "AreaOfTrapezoid" ?.
program:
class AreaOfTrapezoid
{
int b1,b2,h;
float a;
void set()
{
b1=2;
b2=3;
h=4;
}
void areaOfTrapezoid()
{
a=b1+b2*h*0.5;
}
void display()
{
System.out.println("value of b1="+b1);
System.out.println("value of b2="+b2);
System.out.println("areaoftrapezoid="+a);
}
}
class AreaDemo
{
public static void main(String args[])
{
AreaOfTrapezoid a=new AreaOfTrapezoid();
a.set();
a.areaOfTrapezoid();
a.display();
}
}
Q. write a Java program which will display the "SlopeOfLine" ?.
Program :
class SlopeOfLine
{
int x1,x2,y1,y2;
float m;
void set()
{
x1=1;
x2=2;
y1=2;
y2=4;
}
void slopeOfLine()
{
m=y2-y1/x2-x1;
}
void display()
{
System.out.println("value of x1="+x1);
System.out.println("value of x2="+x2);
System.out.println("value of y1="+y1);
System.out.println("value of y2="+y2);
System.out.println("slopeofline="+m);
}
}
class SlopeDemo
{
public static void main(String args[])
{
SlopeOfLine s= new SlopeOfLine();
s.set();
s.slopeOfLine();
s.display();
}
}
Q. write a Java program which will display the "Volume of a Cone" ?.
Program :
class VolumeOfCone
{
int b,h;
float v;
void set()
{
b=2;
h=3;
}
void volumeOfCone()
{
v=b*h*0.3;
}
void display()
{
System.out.println("value of b="+b);
System.out.println("value of h="+h);
System.out.println("volume of a cone="+v);
}
}
class ConeDemo
{
public static void main(String args[])
{
VolumeOfCone= new VolumeOfCone();
s.set();
s.volumeOfCone();
s.display();
}
}
class VolumeOfCone
{
int b,h;
float v;
void set()
{
b=2;
h=3;
}
void volumeOfCone()
{
v=b*h*0.3;
}
void display()
{
System.out.println("value of b="+b);
System.out.println("value of h="+h);
System.out.println("volume of a cone="+v);
}
}
class ConeDemo
{
public static void main(String args[])
{
VolumeOfCone= new VolumeOfCone();
s.set();
s.volumeOfCone();
s.display();
}
}
Q. write a Java program which will display the "SurfaceAreaOfCyclinder" ?.
Program :
class SurfaceAreaOfCyclinder
{
int r,h;
float pi,s;
void set()
{
r=2;
h=3;
pi=3.14f;
}
void surfaceAreaOfCyclinder()
{
s=2*pi*r*h;
}
void display()
{
System.out.println("value of r="+r);
System.out.println("value of h="+h);
System.out.println("SurfaceAreaOfCyclinder="+s);
}
}
class AreaOfCyclinder
{
public static void main(String args[])
{
SurfaceAreaOfCyclinder= new SurfaceAreaOfCyclinder();
s.set();
s.surfaceAreaOfCyclinder();
s.display();
}
}
class SurfaceAreaOfCyclinder
{
int r,h;
float pi,s;
void set()
{
r=2;
h=3;
pi=3.14f;
}
void surfaceAreaOfCyclinder()
{
s=2*pi*r*h;
}
void display()
{
System.out.println("value of r="+r);
System.out.println("value of h="+h);
System.out.println("SurfaceAreaOfCyclinder="+s);
}
}
class AreaOfCyclinder
{
public static void main(String args[])
{
SurfaceAreaOfCyclinder= new SurfaceAreaOfCyclinder();
s.set();
s.surfaceAreaOfCyclinder();
s.display();
}
}
Compile and set the class path in java:
Now we will discuss about on how to set the class path for compiling & executing the java program. The given below points are understand that how to compile the java program piratically.
1) Firstly we can create a folder/directory in any drive option of my computer and save your program in that folder . Here i will create a folder in D: (Drive) with name as Java .
Here i save the program with file name in that folder with extension called ".java".
Here i save the program with file name in that folder with extension called ".java".
Example:- D: Java ➡️ FirstProgram.java (File name)
2) Compile the Java program like this .
Example:- D://cd java ➡️ D://java> Javac FirstProgram.java (file name) ↵
3) Error will occur like this "Javac is not recognized as internally and externally command ".
4) Now, we have to set the path for javac & java tools .
Example :- D:// java>set path="C:\Program Files\Java\ jdk 1.8.0_144\bin";
5) Again we recompile the java program .
Example :- D:// java> javac FirstProgram.java↵
6) To ensure that FirstProgram.class file name is generated when we compile the java program .
7) Run the java program .
Example:- D://java>java FirstProgram ↵
When the above statements is executed the following operations will be performed.
5) Again we recompile the java program .
Example :- D:// java> javac FirstProgram.java↵
6) To ensure that FirstProgram.class file name is generated when we compile the java program .
7) Run the java program .
Example:- D://java>java FirstProgram ↵
When the above statements is executed the following operations will be performed.
- ClassLoader subsystem loads/transfer FirstProgram.class file in to "MainMemmory" .
- JVM takes loaded classes (FirstProgram).
- JVM looks for main method in the form of Array Of Object Of String class "(String args [])" .
- JVM calls main (String args[]) with respect to the loaded classes as FirstProgram.java
- Finally we get the output of the program.
No comments:
Post a Comment
Thanks for visiting my site..